Polymorphism
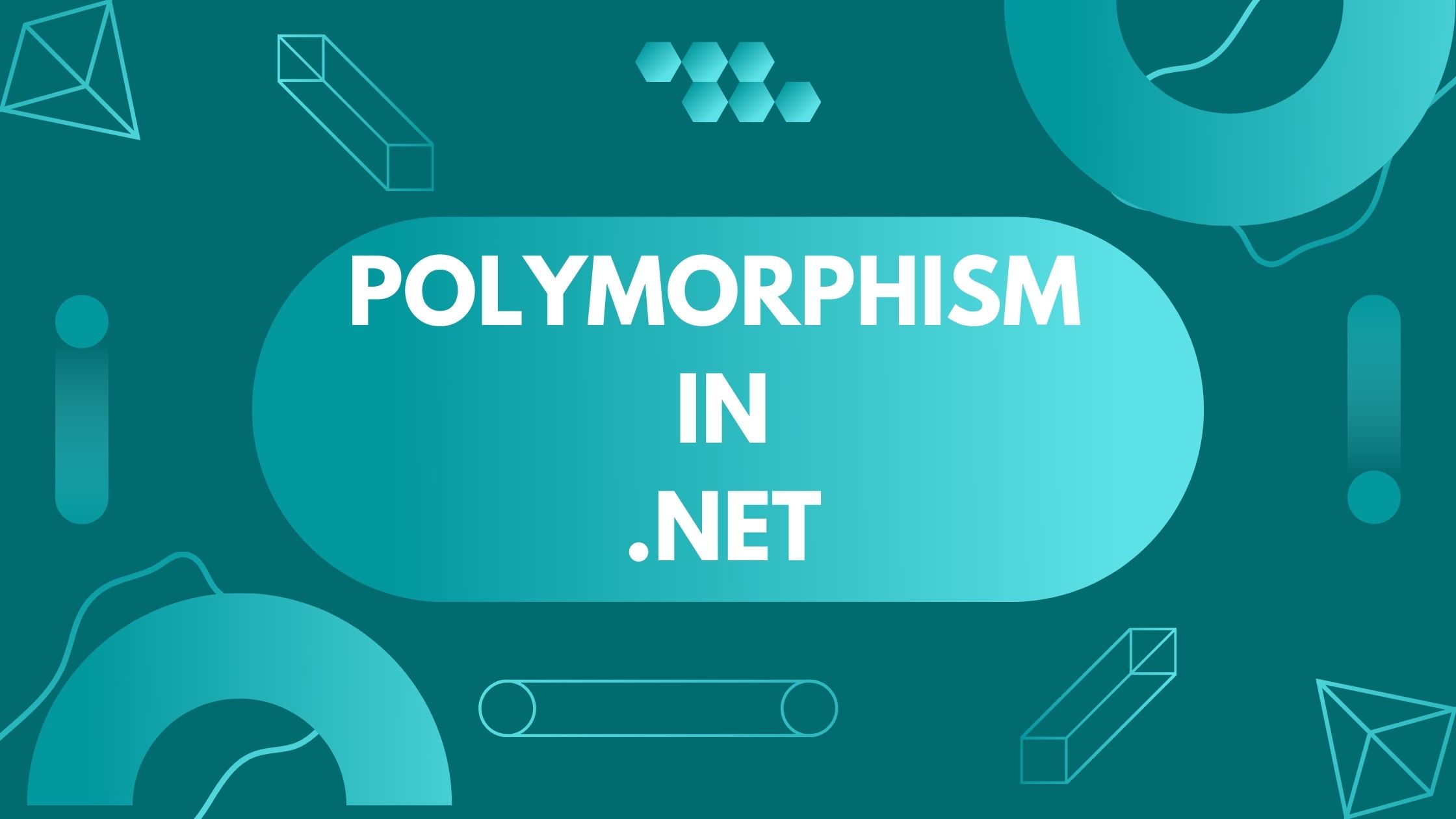
Polymorphism is a Greek word, meaning “one name many forms”. In other words, one object has many forms or has one name with multiple functionalities. “Poly” means many and “morph” means forms. It means ability to take more than one form. The same function will show different behaviour, when we passsed different type of value or different no. of value. So in different words behaving in different ways depending upon input receive is known as polymorphism. Polymorphism provides the ability to a class to have multiple implementations with the same name. It is one of the core principles of Object Oriented Programming after encapsulation and inheritance.
Polymorphism Types:
In C#, there are two types of polymorphism:
- Static / Compile Time Polymorphism(Early Binding).
- Dynamic/Run Time Polymorphism(Late Binding).
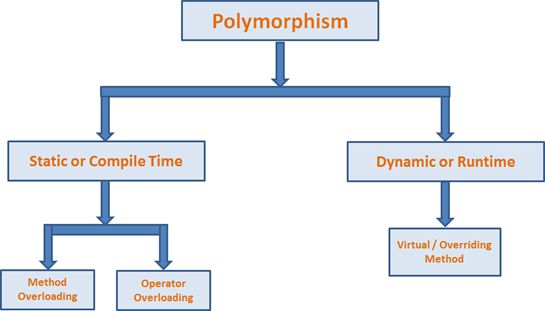
1. Static/Compile Time Ploymorphism:
It is also known as Early Binding. Method overloading is an example of Static Polymorphism. In overloading, the method / function has a same name but different signatures. It is also known as Compile Time Polymorphism because the decision of which method is to be called is made at compile time.
The object of class recognizes which method to be executed for particular method call at the time of compilation and bind method call with that method definition.Method Overloading is the example of static polymorphism.
Method Overloading:
In case of method overloading each method will have different signatur and based on that method call we can easily recognized which method matches with method definition.
public class TestData
{
public int Add(int a, int b, int c)
{
return a+b+c;
}
public int Add(double a, int b)
{
return a + b;
}
}
class Program
{
static void Main(string[] args)
{
TestData dataClass = new TestData();
int add2 = dataClass.Add(45, 34, 67);
int add1 = dataClass.Add(23.05, 34);
}
}
2. Dynamic/Run Time Ploymorphism:
Dynamic / runtime polymorphism is also known as late binding. Here, the method name and the method signature (number of parameters and parameter type must be the same and may have a different implementation). Method overriding is an example of dynamic polymorphism.
In this case, object of class recognizes which method is executed for particular method call at run-time but not at compile-time is called dynamic polymorphism.
Method Overriding:
class Class1
{
publicvirtualvoid show(){
Console.WriteLine(“Super class show method”);
}
}
class Class2 : Class1
{
publicoverridevoid show()
{
Console.WriteLine(“Sub class override show method”);
}
}
class Program
{
static void Main(string[] args)
{
Class2 obj = new Class2();
obj.show();
Console.ReadKey();
}
}
In the above method call, the compiler will search show() method definition in the class “Class2” as the reference type of obj variable is Class2. As it found the method definition in the class “Class2”, so the compilation is successful. At run time, the CLR will check the method implementation in class “Class2” as the reference variable obj holds the Class2 object reference. And it will execute the method from that class.