Interface In C#
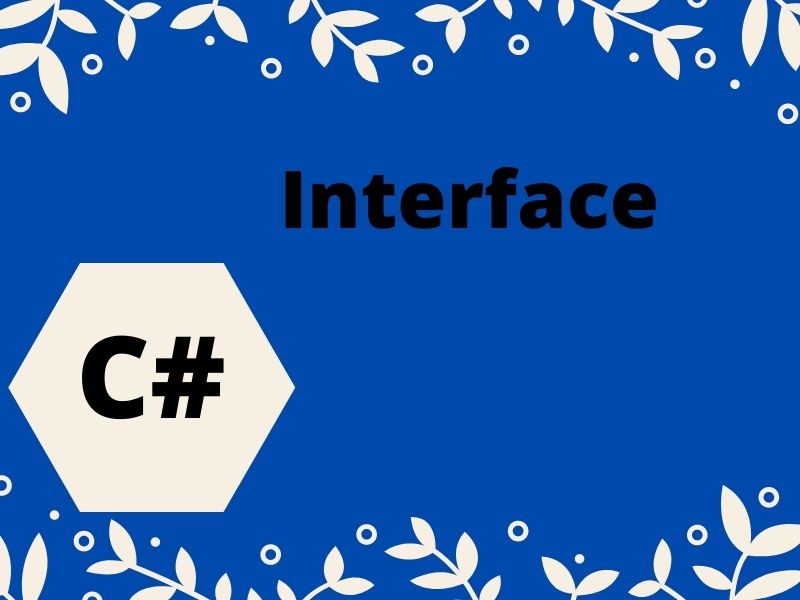
Interface is also a user-defined type like a class but contain only “Abstract Member” in it and all those abstract members should be implemented by a child class of the interface.
Non-Abstract Class :- Contains only non-abstract / concrete members.
Abstract Class :- Contains both non-abstract / concrete and abstract members.
Interface :- Contains only abstract concrete members.
Just like a class can have another class as its parent, it can also have an interface as its parent but the main difference is if a class is a parent we call it as inheriting whereas if an interface is a parent we call it as implementing.
Inheritance is divided into 2 categories:
- Implementation Inheritance
- Interface Inheritance
If a class is inheriting from another class we call it as Implementation Inheritance whereas if a class is implementing an interface we call it as Inetrface Inheritance. Implementation Inheritance provide re-usability because by inheriting from a class we can consume members of a parent in child class whereas Interface Inheritance dosen’t provide ant re-usability because in this case we need to implement abstract members of a parent in child class, but not consume.
Syntax Of Interface:
[<modifiers>] interface <Name>
{
-Abstract member declarations.
}
- We can’t declare any fields under an interface.
- Default scope for members of an interface is public whereas it is private in case of a class.
- Every member of an interface is by default abstract, so we again don’t require using abstract modifier on it.
- Just like a class can inherit from another class, an interface can also inherit from another interface, but not from a class.
Example on Interface:
interface IDemoInterface
{
void SampleMethod();
}
class ImplementationClass : IDemoInterface
{
// Explicit interface member implementation:
void ISampleInterface.SampleMethod()
{
// Method implementation.
}
static void Main()
{
// Declare an interface instance.
IDemoInterface obj = new ImplementationClass();
// Call the member.
obj.SampleMethod();
}
}
Difference Between Inerface And Abstract Class”
An abstract class is a way to achieve the abstraction in C#. An Abstract class is never intended to be instantiated directly. This class must contain at least one abstract method, which is marked by the keyword or modifier abstract in the class definition. The Abstract classes are typically used to define a base class in the class hierarchy.