C# Inheritance
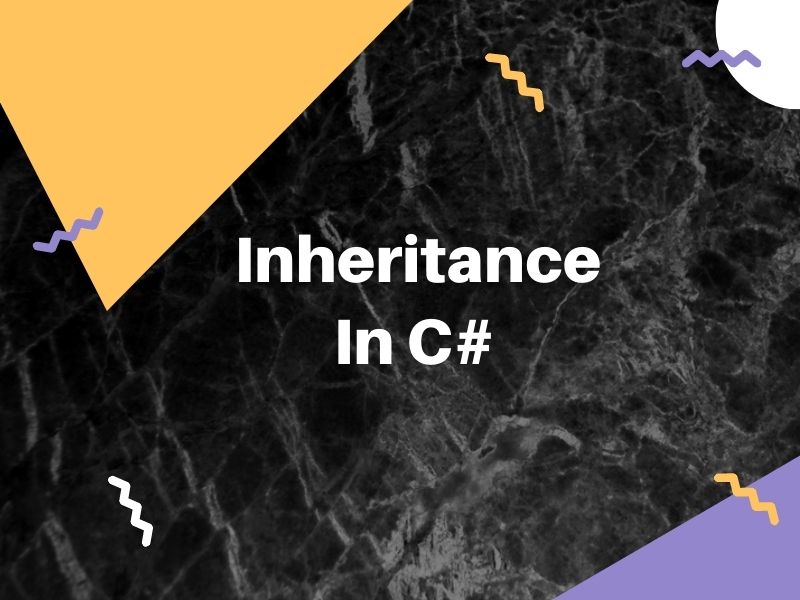
What Is Inheritance:
Inheritance is a feature of object-oriented programming languages that allows derived classes to inherit or override the functionality (data and behavior) of a base class.
The method of constructing one class from another class is called Inheritance. The derived class inherites all the properties and methods from base class and add its own methods also. Base class is also known as parent class and derived class is also known as child class.
Parent Class: In Inheritance, the class whose features are inherited is known as parent class(or a generalized class, superclass, or base class).
Child Class: The class that inherits the existing class is known as child class(or a specialized class, derived class, extended class, or subclass).
Syntax Of Inheritance:
Inheritance uses “:” colon to make a relationship between parent class and child class as you can see in the below syntax of code.
<AccessModifier> class<BaseClassName>
{ // Base class code
}
<AccessModifier> class<DerivedClassName> : <BaseClassName>
{
// Derived class code
}
Advantages Of Inheritance:
1.Code Reusability:
Public and protected methods can be used in derived class.
2.Extensibility:
Base class can be extented as per the logic in derived class
Types Of Inheritance:
Thera are five types of inheritance.
- Single Inheritance
- Multilevel Inheritance
- Multiple Inheritance
- Hierarchical Inheritance
- Hybrid Inheritance
1. Single Inheritance:
In single inheritance, a derived class inherit the features or behavior of one base class.
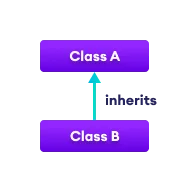
public class A
{
// Code Implementation
}
public classB: A
{
// Code Implementation
}
class A serves as a base class for the derived class B.
2. Multilevel Inheritance:
In Multilevel Inheritance, one class inherits another class which is further inherited by another class. Inheritance in C# is transitive so the last derived class acquires all the members of all its base classes.
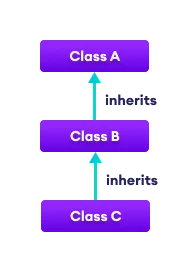
public class A
{
// Code Implementation}
public class B : A
{
// Code Implementation
}
public class C : B
{
// Code Implementation
}
For example, if class “C” is derived from class “B”, and further class “B” is derived from class “A”, then class “C” will able to inherits the members declared in both class “B” and class “A”.
The following image is an example of multi-level inheritance in C#
3.Multiple Inheritance:
A derived class can have more than one base class and access their functionality.
C# does not support multiple inheritance because of diamond problem. The diamond problem occurs in multiple inheritance.
In below diagram, I have Class C which is derived from Class A and Class B then is Class C calls a methods from A and B then ambugity problem occurs, that’s why C# does not support multiple inheritance.But, we can achived multiple inheritance through Interface.
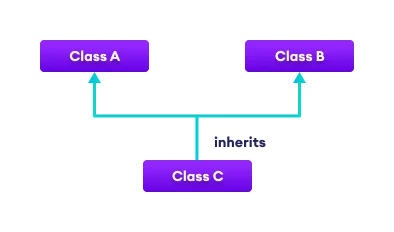
interface A
{
// Code implementation
}
interface B
{
// Code implementation
}
// Derived class
class C : A,B
{
// Code Implementation
}
4. Hierarchical Inheritance:
In Hierarchical Inheritance, one class serves as a base class for more than one derived classes.
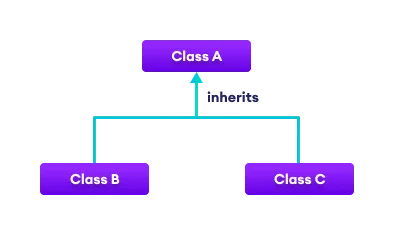
In the following example, class A refers as a base class for the derived class B, class C, and class D.
//Base class
class A
{
// Code implementation
}
// Derived class
class B :A
{
// Code implementation
}
// Derived class
class C : A
{
// Code Implementation
}
// Derived class
class D :A
{
// Code Implementation
}
5. Hybrid Inheritance:
In C#, the hybrid inheritance is not supported with classes, but hybrid inheritance can be achieve through interfaces only. Actually, hybrid inheritance is a mix of multiple types of inheritance.
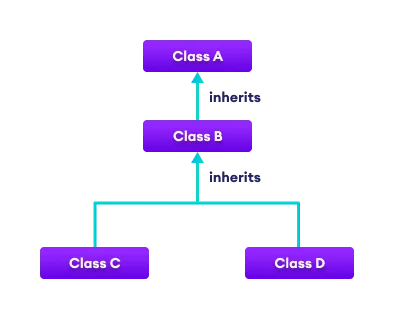
Important Points Of Inheritance:
- In C #, it does not support inheritance, but it can be inherited through interfaces.
- The object class is the base class of all subclasses. All classes in C # are implicitly derived from the Object class.
- C # only supports single inheritance, but we can implement multiple inheritance through interfaces.
- A child class can’t inherit the private members of the its parent class until or unless the base class doesn’t have public properties to access the private fields.
- A subclass can inherit all the members of its base class, except the constructor, because the constructor is not a data member of the class. But the constructor of the base class can be called from the subclass.