Abstarction In C#
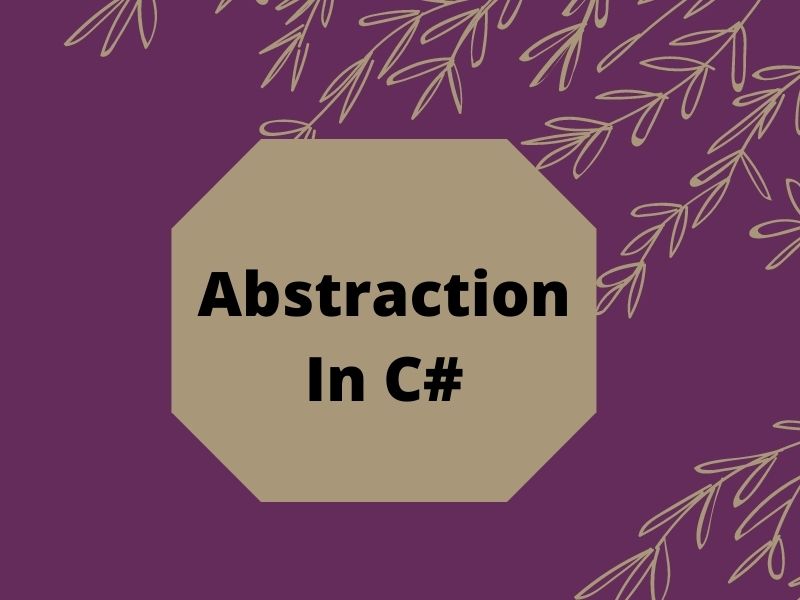
The Abstraction in C# is one of the fundamental OOPs principles which acts as a supporting principle. Abstraction means to abstract the necessary data and avoid all remaing data. It catch only necessary data and avoid the implementation details.
The process of representing the essential features without including the background details is called Abstraction. In simple words, we can say that it is a process of defining a class by providing necessary details to call the object operations (i.e. methods) by hiding or removing its implementation details is called abstraction in C#. It means we need to expose what is necessary and compulsory and we need to hide the unnecessary things from the outside world. In C# we can hide the member of a class by using private access modifiers.
Real-time Example of Abstraction:
Let us understand this with a car example. As we know a car is made of many things, such as the name of the car, the color of the car, gear, breaks, steering, silencer, the battery of the car, engine of the car, etc. Now you want to ride a car. So to ride a car what are the things you should know. The things a car driver should know are as follows.
- Name of the Car
- The color of the Car
- Gear
- Break
- Steering
So these are the things that should be exposed and know by the car driver before riding the car. The things which should be hidden to a Car rider as are follows
- The engine of the car
- Diesel Engine
- Silencer
- How battery work
So these are the things which should be hidden from a car driver.
public class Car
{
private string _CarName = “Honda City”;
private string _CarColur = “Black”;
public string CarName
{
set{ _CarName = value; }
get{return _CarName; }
}
public string CarColur
{
set{ _CarColur = value; }
get{return _CarColur; }
}
public void Steering()
{
Console.WriteLine(“Streering of the Car”);
}
public void Brakes()
{
Console.WriteLine(“Brakes of the Car”);
}
public void Gear()
{
Console.WriteLine(“Gear of the Car”);
}
private void CarEngine()
{
Console.WriteLine(“Engine of the Car”);
}
private void DiesalEngine()
{
Console.WriteLine(“DiesalEngine of the Car”);
}
privatevoid Silencer()
{
Console.WriteLine(“Silencer of the Car”);
}
}
As shown in the above example, you can see that the necessary methods and properties are exposed by using the “public” access modifier whereas the unnecessary methods and properties hidden from outside the world by using the “private” access modifier as shown in the below image.
As you can see in the above example, the methods and properties which we want to expose to the outside world are created using the public access specifier. Now from outside the class, we can create the object of this Car class and can access the above methods and properties and the methods and variables which don’t want to expose to the outside world are created using the private access modifier. Now from outside the class, we can create the instance of the Car class but we cannot access the above methods and variables.
Consuming the Car Class:
Let us create an instance of the Car class within the Main method of the Program class and then let’s try to invoke the public and private members of the Car class.
public class Program
{
public static void Main()
{
//Creating an instance of Car
Car CarObject = new Car();
//Accessing the Public Properties and methods
string CarName = CarObject.CarName;
string CarColur = CarObject.CarColur;
CarObject.Brakes();
CarObject.Gear();
CarObject.Steering();
//Try to access the private variables and methods
//Compiler Error, ‘Car._CarName’ is inaccessible due to its protection level CarObject._CarName;
//Compiler Error, ‘Car.CarEngine’ is inaccessible due to its protection level CarObject.CarEngine();
}
}
As you can see, we can access the public members of the Car class using the Car instance. But while we are accessing the private members of the Car class using the same Car instance, we get Compiler Error. Hence, this proofs that, we have exposed the necessary methods and properties to outside the world or class by hiding the unnecessary members of the class by using the Abstraction in C#
Difference between Abstraction and Encapsulation:
Encapsulation is the process of hiding irrelevant data from the user or you can say Encapsulation is used to protect the data. For example, whenever we buy a mobile, we can never see how the internal circuit board works. We are also not interested to know how the digital signal converts into the analog signal and vice versa. So from a Mobile user’s point of view, these are some irrelevant pieces of information, This is the reason why they are encapsulated inside a cabinet.
In C# programming, we will do the same thing. We will create a cabinet and keep all the irrelevant information that should not be exposed to the user of the class.
Coming to abstraction in C#, It is just the opposite of Encapsulation. What it means, it is a mechanism that will show only the relevant information to the user. If we consider the same mobile example. Whenever we buy a mobile phone, we can see and use many different types of functionalities such as a camera, calling function, recording function, mp3 player, multimedia, etc. This is nothing but an example of abstraction in C#. The reason is we are only seeing the relevant information instead of their internal work.